Monday, March 9, 2015
In the last blog we just selected the Products entities from the NorthwindEntities with this Linq Query.
It gets the job done but, the GridView displays the CategoryID and SupplierID as integers, which is not very useful to your users. Plus, we don't want to display the ProductID.
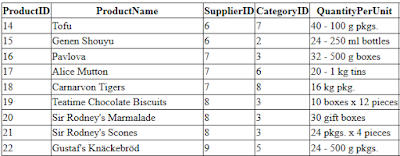
In this blog we are going to refine the LINQ query so that the GridView only display the columns that we want to display with Projection and Anonymous Types. Projection is to transform an object into a new form with only the properties that you want in the new form. In our case we want to transform the Products DataSet to a new form that only returns the data that we want.
The resulting GridView after projection is looks like this.
Notice that the ID column is hidden and the Category and Supplier shows the actual Category name and the Supplier name.
Here are the steps:
1. Open up the project that you've completed on the last blog.
2. Change the ID of the GridView to ProductsGrid 3. Comment out the code in the Page_Load method
4. In the Default.aspx.cs code behind file add the following method
The code above uses LINQ to query the Products entity, and then joins the Categories and the Suppliers entities to get the "Category Name", and Supplier's "Company Name" respectively. We assign the Products.DataKeyNames property in the GridView control to an array of string values with the "ID" field from the LINQ query. The "select new" part of the query creates a new anonymous type on the fly with only the columns that we want from the resulting query. Then we bind the query to the ProductsGrid.DataSource.
4. In the Page_Load method, call the BindProductsGrid() method
6. To hide the "ID" column we have to create an event handler RowDataBound on the ProductsGrid to make the "ID" invisible while the ProductsGrid is binding the data to the rows. 7. To create the event handler RowDataBound click the ProductsGrid, then in the Properties window double click the event handler RowDataBound
8. A ProductsGrid_RowDataBound method will be created in the code behind, since the "ID" column is the first column we can just hide the first column of the ProductsGrid with the following code
9. Press Ctrl + F5 to run the application and you will see that the "ID" column is hidden
Blogs in the Entity Framework Series:
var query = from prod in nwctx.Products select prod;
It gets the job done but, the GridView displays the CategoryID and SupplierID as integers, which is not very useful to your users. Plus, we don't want to display the ProductID.
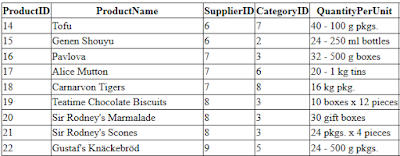
In this blog we are going to refine the LINQ query so that the GridView only display the columns that we want to display with Projection and Anonymous Types. Projection is to transform an object into a new form with only the properties that you want in the new form. In our case we want to transform the Products DataSet to a new form that only returns the data that we want.
The resulting GridView after projection is looks like this.
Notice that the ID column is hidden and the Category and Supplier shows the actual Category name and the Supplier name.
Here are the steps:
1. Open up the project that you've completed on the last blog.
2. Change the ID of the GridView to ProductsGrid 3. Comment out the code in the Page_Load method
4. In the Default.aspx.cs code behind file add the following method
protected void BindProductsGrid() { using(NorthwindEntities ntcx = new NorthwindEntities()) { var query = from prod in ntcx.Products join cat in ntcx.Categories on prod.CategoryID equals cat.CategoryID join sup in ntcx.Suppliers on prod.SupplierID equals sup.SupplierID select new { ID = prod.ProductID, Name = prod.ProductName, Price = prod.UnitPrice, QuantityPerUnit = prod.QuantityPerUnit, Category = cat.CategoryName, Supllier = sup.CompanyName }; string[] dataKeyNames = new string[] { "ID" }; ProductsGrid.DataSource = query.ToList(); ProductsGrid.DataKeyNames = dataKeyNames; ProductsGrid.DataBind(); } }
The code above uses LINQ to query the Products entity, and then joins the Categories and the Suppliers entities to get the "Category Name", and Supplier's "Company Name" respectively. We assign the Products.DataKeyNames property in the GridView control to an array of string values with the "ID" field from the LINQ query. The "select new" part of the query creates a new anonymous type on the fly with only the columns that we want from the resulting query. Then we bind the query to the ProductsGrid.DataSource.
4. In the Page_Load method, call the BindProductsGrid() method
protected void Page_Load(object sender, EventArgs e) { BindProductsGrid(); }5. Press Ctrl+F5 to run the application, you will see that ProductsGrid is populated by the fields in the projection type. However, since the ID field is part of the anonymous type it shows up in the ProductsGrid
6. To hide the "ID" column we have to create an event handler RowDataBound on the ProductsGrid to make the "ID" invisible while the ProductsGrid is binding the data to the rows. 7. To create the event handler RowDataBound click the ProductsGrid, then in the Properties window double click the event handler RowDataBound
8. A ProductsGrid_RowDataBound method will be created in the code behind, since the "ID" column is the first column we can just hide the first column of the ProductsGrid with the following code
protected void ProductsGrid_RowDataBound(object sender, GridViewRowEventArgs e) { e.Row.Cells[0].Visible = false; }
9. Press Ctrl + F5 to run the application and you will see that the "ID" column is hidden
Blogs in the Entity Framework Series:
Subscribe to:
Post Comments (Atom)
Search This Blog
Tags
Web Development
Linux
Javascript
DATA
CentOS
ASPNET
SQL Server
Cloud Computing
ASP.NET Core
ASP.NET MVC
SQL
Virtualization
AWS
Database
ADO.NET
AngularJS
C#
CSS
EC2
Iaas
System Administrator
Azure
Computer Programming
JQuery
Coding
ASP.NET MVC 5
Entity Framework Core
Web Design
Infrastructure
Networking
Visual Studio
Errors
T-SQL
Ubuntu
Stored Procedures
ACME Bank
Bootstrap
Computer Networking
Entity Framework
Load Balancer
MongoDB
NoSQL
Node.js
Oracle
VirtualBox
Container
Docker
Fedora
Java
Source Control
git
ExpressJS
MySQL
NuGet
Blogger
Blogging
Bower.js
Data Science
JSON
JavaEE
Web Api
DBMS
DevOps
HTML5
MVC
SPA
Storage
github
AJAX
Big Data
Design Pattern
Eclipse IDE
Elastic IP
GIMP
Graphics Design
Heroku
Linux Mint
Postman
R
SSL
Security
Visual Studio Code
ASP.NET MVC 4
CLI
Linux Commands
Powershell
Python
Server
Software Development
Subnets
Telerik
VPC
Windows Server 2016
angular-seed
font-awesome
log4net
servlets
tomcat
AWS CloudWatch
Active Directory
Angular
Blockchain
Collections
Compatibility
Cryptocurrency
DIgital Life
DNS
Downloads
Google Blogger
Google Chrome
Google Fonts
Hadoop
IAM
KnockoutJS
LINQ
Linux Performance
Logging
Mobile-First
Open Source
Prototype
R Programming
Responsive
Route 53
S3
SELinux
Software
Unix
View
Web Forms
WildFly
XML
cshtml
githu
Aivivu chuyên vé máy bay, tham khảo
ReplyDeletesăn vé máy bay giá rẻ đi Mỹ
ve may bay tu my ve vietnam
chuyến bay từ frankfurt đến hà nội
chuyến bay nhật bản về việt nam
dat ve may bay tu han quoc ve viet nam
lịch bay từ canada về việt nam
Chi phí cho chuyên gia nước ngoài