Friday, July 18, 2014
The RadioButtonList displays a collection of radio buttons on a web page. There can only be one selection in a group of choices at a time. In this tutorial we will create a RadioButtonList and then bind it to the Categories table of the Northwind database using a DataTable.
To create a RadioButtonList control do the following:
1. Select the "RadioButtonList" control under the "Standard" control in the "Toolbox" pane on the left.
2. Drag the RadioButtonList control to a design surface
3. In the source code of the .aspx page make sure "AutoPostBack" is set to true, the source code should look like this
4. Create a connection string in the Web.Config file
The code above creates new DataTable call dtCategories, then it connects to the database using the "NorthwindConnectionString", and queries the CategoryID, and CategoryName in the Categories table in the Northwind database. The query results are then stored in the DataTable. The next step is to use the data in the dtCategories DataTable to populate the RadioButtonList1 control on our .aspx page. The following lines are used to bind the dtCategories to the RadioButtonList1 control. It also
selects "Seafood" by default
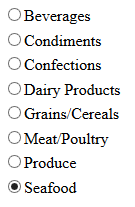
7. Call the BindRadioButtonList method in the Page_Load method, you can call on the first page load only
Note: If AutoPoskBack is not set to true in the RadioButtonList1 control the RadioButtonList1_SelectedIndexChanged event handler would not have worked because there will not be a post back to the server when the user changes his/her selection.
To create a RadioButtonList control do the following:
1. Select the "RadioButtonList" control under the "Standard" control in the "Toolbox" pane on the left.
2. Drag the RadioButtonList control to a design surface
3. In the source code of the .aspx page make sure "AutoPostBack" is set to true, the source code should look like this
<asp:RadioButtonList ID="RadioButtonList1" runat="server" AutoPostBack="true"> </asp:RadioButtonList>
4. Create a connection string in the Web.Config file
<connectionStrings> <add name="NorthwindConnectionString" connectionString="Data Source=(local); Initial Catalog=Northwind;Integrated Security=True" providerName="System.Data.SqlClient"/> </connectionStrings>5. In the code behind page(.cs) page of the .aspx page, type in the following lines at the top to use the following libraries
using System.Web.Configuration; using System.Data.SqlClient; using System.Data;6. In the code behind file add the BindRadioButtonList method
protected void BindRadioButtonList() { DataTable dtCategories = new DataTable(); string connectString = WebConfigurationManager.ConnectionStrings["NorthwindConnectionString"]. ConnectionString; using (SqlConnection conn = new SqlConnection(connectString)) { SqlCommand cmd = new SqlCommand("SELECT CategoryID,CategoryName FROM Categories", conn); conn.Open(); SqlDataAdapter adapter = new SqlDataAdapter(cmd); adapter.Fill(dtCategories); //used to set the RadioButtonList1.DataSource to dtCategories RadioButtonList1.DataSource = dtCategories; //set the text field of the RadioButtonList1 to the "CategoryName" value in the database RadioButtonList1.DataTextField = dtCategories.Columns["CategoryName"].ToString(); //set the value field of the RadioButtonList1 to the "CategoryID" value in the database RadioButtonList1.DataValueField = dtCategories.Columns["CategoryID"].ToString(); RadioButtonList1.DataBind(); RadioButtonList1.Items.FindByText("Seafood").Selected = true; } }
The code above creates new DataTable call dtCategories, then it connects to the database using the "NorthwindConnectionString", and queries the CategoryID, and CategoryName in the Categories table in the Northwind database. The query results are then stored in the DataTable. The next step is to use the data in the dtCategories DataTable to populate the RadioButtonList1 control on our .aspx page. The following lines are used to bind the dtCategories to the RadioButtonList1 control. It also
selects "Seafood" by default
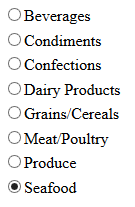
7. Call the BindRadioButtonList method in the Page_Load method, you can call on the first page load only
protected void Page_Load(object sender, EventArgs e) { if(!Page.IsPostBack) BindRadioButtonList(); }
8. Double click on the RadioButtonList1 control in the design surface, the RadioButtonList1_SelectedIndexChanged method will automatically be generated for you in the code behind file In the RadioButtonList1_SelectedIndexChanged method type in the following to display the item the user has selected
protected void RadioButtonList1_SelectedIndexChanged(object sender, EventArgs e) { Response.Write("Selected button " + RadioButtonList1.SelectedItem.Text); }
Note: If AutoPoskBack is not set to true in the RadioButtonList1 control the RadioButtonList1_SelectedIndexChanged event handler would not have worked because there will not be a post back to the server when the user changes his/her selection.
Subscribe to:
Post Comments (Atom)
Search This Blog
Tags
Web Development
Linux
Javascript
DATA
CentOS
ASPNET
SQL Server
Cloud Computing
ASP.NET Core
ASP.NET MVC
SQL
Virtualization
AWS
Database
ADO.NET
AngularJS
C#
CSS
EC2
Iaas
System Administrator
Azure
Computer Programming
JQuery
Coding
ASP.NET MVC 5
Entity Framework Core
Web Design
Infrastructure
Networking
Visual Studio
Errors
T-SQL
Ubuntu
Stored Procedures
ACME Bank
Bootstrap
Computer Networking
Entity Framework
Load Balancer
MongoDB
NoSQL
Node.js
Oracle
VirtualBox
Container
Docker
Fedora
Java
Source Control
git
ExpressJS
MySQL
NuGet
Blogger
Blogging
Bower.js
Data Science
JSON
JavaEE
Web Api
DBMS
DevOps
HTML5
MVC
SPA
Storage
github
AJAX
Big Data
Design Pattern
Eclipse IDE
Elastic IP
GIMP
Graphics Design
Heroku
Linux Mint
Postman
R
SSL
Security
Visual Studio Code
ASP.NET MVC 4
CLI
Linux Commands
Powershell
Python
Server
Software Development
Subnets
Telerik
VPC
Windows Server 2016
angular-seed
font-awesome
log4net
servlets
tomcat
AWS CloudWatch
Active Directory
Angular
Blockchain
Collections
Compatibility
Cryptocurrency
DIgital Life
DNS
Downloads
Google Blogger
Google Chrome
Google Fonts
Hadoop
IAM
KnockoutJS
LINQ
Linux Performance
Logging
Mobile-First
Open Source
Prototype
R Programming
Responsive
Route 53
S3
SELinux
Software
Unix
View
Web Forms
WildFly
XML
cshtml
githu
Very Nice blog for learning new things, thanks for such beautiful blog.
ReplyDeletesee here
play roulette online free at Potawatomi Hotel & Casino is an easy game to understand, and it's even easier to play.
Delete