Thursday, July 17, 2014
If you work on the DropDownList control, eventually you will be asked to populate some sort of data grid based on the selection on the list. The most common control you have to populate is the GridView control. This tutorial builds from part 1 and part 2 about DropDownList control. So make sure you go through those blogs before you start this one. In this tutorial we will learn how to populate the GridView via a DropDownList selection.
1. Select the "GridView" control from the "Toolbox" pane on the left
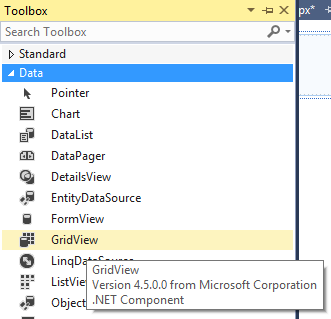
2. Drag the GridView control to a design surface
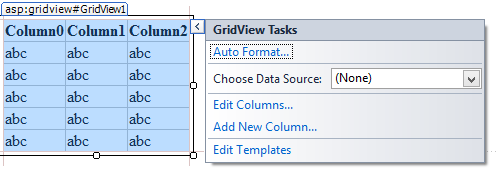
3. Enable the "AutoPostBack" attribute on the "DropDownList1" control, by clicking on the "Source" tab then add the following line
1. Select the "GridView" control from the "Toolbox" pane on the left
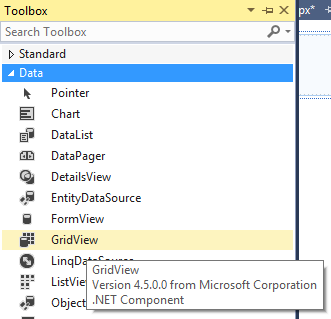
2. Drag the GridView control to a design surface
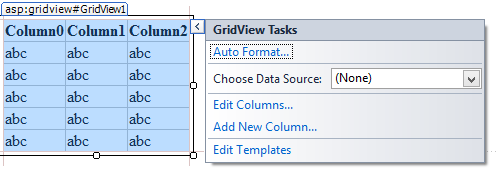
3. Enable the "AutoPostBack" attribute on the "DropDownList1" control, by clicking on the "Source" tab then add the following line
<asp:DropDownList ID="DropDownList1" runat="server" AutoPostBack="true"> </asp:DropDownList>4. In the code behind add the BindGridView method
protected void BindGridView(int catId) { DataTable dtProducts = new DataTable(); string connectString = WebConfigurationManager.ConnectionStrings["NorthwindConnectionString"].ConnectionString; using (SqlConnection conn = new SqlConnection(connectString)) { SqlCommand cmd = new SqlCommand("SELECT DISTINCT ProductID,ProductName,UnitPrice,UnitsInStock " + "FROM Products " + " WHERE Products.CategoryID = @CategoryID", conn); SqlParameter parameter = new SqlParameter(); parameter.ParameterName = "CategoryID"; parameter.SqlDbType = SqlDbType.Int; parameter.Value = catId; cmd.Parameters.Add(parameter); conn.Open(); SqlDataAdapter adapter = new SqlDataAdapter(cmd); adapter.Fill(dtProducts); GridView1.DataSource = dtProducts; GridView1.DataBind(); } }The method above takes an int parameter call "catId" based on the parameter being passed in we query the "Products" table based on the value being passed in. There is a @CategoryID in the SqlCommand, that is a placeholder for an SqlParameter that we will have to create and add to the SqlCommand later. The @CategoryID SqlParamter takes the value of the catId. 5. In the Page_Load method we have to call the BindGridView method
protected void Page_Load(object sender, EventArgs e) { if(!Page.IsPostBack) { BindCategoriesList(); SetDefaultSelectItem("Seafood"); BindGridView(Convert.ToInt32(DropDownList1.SelectedValue)); } else { BindGridView(Convert.ToInt32(DropDownList1.SelectedValue)); } }Notice in the code above we have to call the BindGridView method twice. Once when the page first loads, it will take the default selection which is "Seafood" then on subsequent page loads it will take the user's selection to populate the GridView control.
Related Blogs:
- Bind a DataTable To A DropDownList
- (DropDownList) Setting Default Value on First Page Load
- Use The DropDownList Control To Populate GridView
- Bind DropDownList To A List Of Objects
Subscribe to:
Post Comments (Atom)
Search This Blog
Tags
Web Development
Linux
Javascript
DATA
CentOS
ASPNET
SQL Server
Cloud Computing
ASP.NET Core
ASP.NET MVC
SQL
Virtualization
AWS
Database
ADO.NET
AngularJS
C#
CSS
EC2
Iaas
System Administrator
Azure
Computer Programming
JQuery
Coding
ASP.NET MVC 5
Entity Framework Core
Web Design
Infrastructure
Networking
Visual Studio
Errors
T-SQL
Ubuntu
Stored Procedures
ACME Bank
Bootstrap
Computer Networking
Entity Framework
Load Balancer
MongoDB
NoSQL
Node.js
Oracle
VirtualBox
Container
Docker
Fedora
Java
Source Control
git
ExpressJS
MySQL
NuGet
Blogger
Blogging
Bower.js
Data Science
JSON
JavaEE
Web Api
DBMS
DevOps
HTML5
MVC
SPA
Storage
github
AJAX
Big Data
Design Pattern
Eclipse IDE
Elastic IP
GIMP
Graphics Design
Heroku
Linux Mint
Postman
R
SSL
Security
Visual Studio Code
ASP.NET MVC 4
CLI
Linux Commands
Powershell
Python
Server
Software Development
Subnets
Telerik
VPC
Windows Server 2016
angular-seed
font-awesome
log4net
servlets
tomcat
AWS CloudWatch
Active Directory
Angular
Blockchain
Collections
Compatibility
Cryptocurrency
DIgital Life
DNS
Downloads
Google Blogger
Google Chrome
Google Fonts
Hadoop
IAM
KnockoutJS
LINQ
Linux Performance
Logging
Mobile-First
Open Source
Prototype
R Programming
Responsive
Route 53
S3
SELinux
Software
Unix
View
Web Forms
WildFly
XML
cshtml
githu
No comments:
Post a Comment